How To Make A Youtube Video Downloader With Python: A Step-By-Step Guide
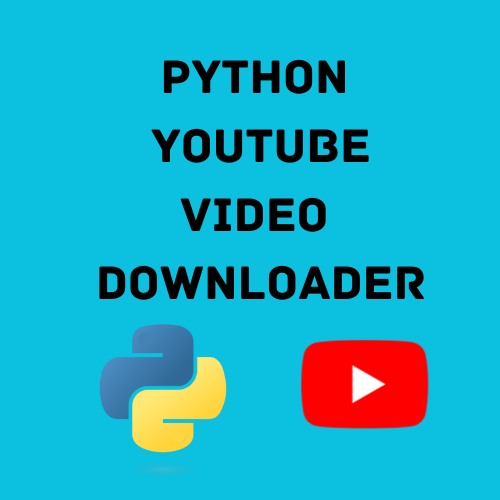
There is a huge variety of videos on YouTube, ranging from entertainment to education and everything in between. You may occasionally wish to download your preferred YouTube videos for viewing offline. This tutorial will walk you through the process of using Python, a well-liked and flexible programming language, to create a YouTube video downloader.
Requirements:
Make sure Python is installed on your system before we start. Python's official website, python.org, offers the most recent version for download. The Pytube library, a small and user-friendly Python library for downloading YouTube videos, will also be used. Use the following command to install it:
pip install pytube
Step 1: Import Necessary Libraries:
First, let's import the required libraries. We will use pytube
for handling YouTube video downloads.
from pytube import YouTube
Step 2: Create a Function for Downloading Videos:
Next, create a function that takes a YouTube video URL as input and downloads the video in the highest available resolution. We'll handle potential errors as well.
def download_video(video_url):
try:
yt = YouTube(video_url)
video = yt.streams.filter(progressive=True, file_extension='mp4').order_by('resolution').desc().first()
video.download()
print("Download completed successfully!")
except Exception as e:
print("Error:", str(e))
In this function, we use the pytube
library to access the YouTube video and download it. We select a video stream with a progressive format and an MP4 file extension for optimal compatibility.
Step 3: Get User Input and Initiate the Download:
Now, let's create a simple user interface where the user can input the YouTube video URL.
if __name__ == "__main__":
video_url = input("Enter the YouTube video URL: ")
download_video(video_url)
In this section, the program prompts the user to enter the YouTube video URL. Once the user inputs the URL, the download_video
function is called with the provided URL, initiating the download process.
Step 4: Run the Script:
Save your Python script with an appropriate name, such as youtube_downloader.py
, and run it using your preferred Python interpreter. When prompted, enter the YouTube video URL you want to download. The script will download the video with the best available resolution and save it in the same directory as your Python script.
Congratulations! You have successfully created a YouTube video downloader using Python and the pytube
library. Remember to use this knowledge responsibly and respect copyright laws and YouTube's terms of service when downloading videos. Happy coding!