Crafting Efficiency: Build Your Own Image Size Compressor With Html, Css, And Javascript
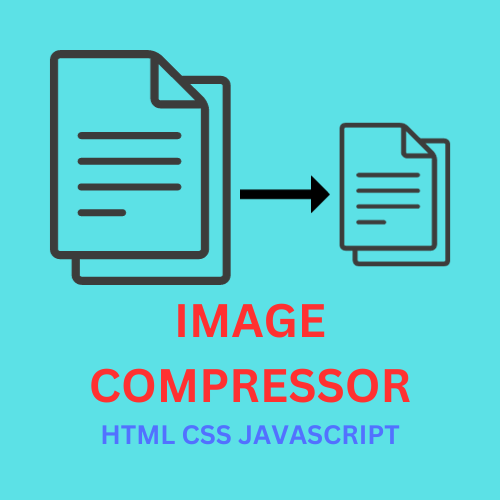
In today's fast-paced digital world, visuals play a pivotal role in capturing attention and conveying messages effectively. However, large image files can significantly slow down websites, affecting user experience and search engine rankings. The solution? An Image Size Compressor – your ticket to a faster, sleeker, and more user-friendly website. In this article, we'll guide you through the process of creating a powerful Image Size Compressor using the dynamic trio of HTML, CSS, and JavaScript. Buckle up and get ready to optimize your web images effortlessly!
1. Setting the Stage:
First things first, let's create the basic structure of our compressor. We'll need an HTML file to set up the user interface, a CSS file for styling, and a JavaScript file to handle the image compression logic. Create these files in the same directory to keep everything organized.
2. Designing the User Interface:
A user-friendly interface is essential for any application. Design a clean and intuitive layout using HTML and CSS. Include elements like an input field for file selection, a compression level slider, and a button to trigger the compression process. Add some creative flair with CSS to make your interface visually appealing and responsive.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Image Size Compressor</title>
</head>
<body>
<div class="container">
<input type="file" id="imageInput" accept="image/*">
<label for="compressionLevel">Compression Level:</label>
<input type="range" id="compressionLevel" min="0" max="1" step="0.1" value="0.5">
<button id="compressButton">Compress Image</button>
<div class="output" id="output"></div>
</div>
<script src="script.js"></script>
</body>
</html>
3. Styling for Appeal:
Add life to your interface with CSS. Style the elements to make them visually engaging, ensuring a seamless user experience. Consider using vibrant colors, smooth transitions, and responsive design principles to make your compressor visually appealing across various devices.
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f1f1f1;
}
.container {
text-align: center;
background-color: #ffffff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
input, label, button {
margin: 10px 0;
}
.output {
margin-top: 20px;
font-weight: bold;
}
4. JavaScript Magic:
Time to add the brains to your compressor. Use JavaScript to handle user interactions, compress the selected image, and display the compressed file size. Utilize the HTML5 Canvas API to perform the image compression.
const imageInput = document.getElementById('imageInput');
const compressionLevel = document.getElementById('compressionLevel');
const compressButton = document.getElementById('compressButton');
const output = document.getElementById('output');
const downloadLink = document.createElement('a');
compressButton.addEventListener('click', () => {
const image = imageInput.files[0];
const reader = new FileReader();
reader.onload = function(event) {
const img = new Image();
img.src = event.target.result;
img.onload = function() {
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
canvas.width = img.width * compressionLevel.value;
canvas.height = img.height * compressionLevel.value;
ctx.drawImage(img, 0, 0, canvas.width, canvas.height);
canvas.toBlob((blob) => {
const compressedImage = new File([blob], 'compressed_image.jpg', { type: 'image/jpeg' });
const compressedSize = (compressedImage.size / 1024).toFixed(2);
output.textContent = `Compressed Image Size: ${compressedSize} KB`;
// Create download link
const url = window.URL.createObjectURL(compressedImage);
downloadLink.href = url;
downloadLink.download = 'compressed_image.jpg';
downloadLink.textContent = 'Download Compressed Image';
// Append download link to the output div
output.appendChild(downloadLink);
}, 'image/jpeg', 0.7);
};
};
reader.readAsDataURL(image);
});
5. Testing Your Image Size Compressor:
Open your HTML file in a web browser, and voilà! You now have a functional Image Size Compressor at your fingertips. Select an image, adjust the compression level, and watch as your image size reduces while retaining its quality.
By following these simple steps, you've empowered yourself to optimize your website's performance with a custom-built Image Size Compressor. Enhance user experience, boost your site's speed, and make a lasting impression with your visually optimized web content. Happy compressing!