Building A Basic Blog With Python Django
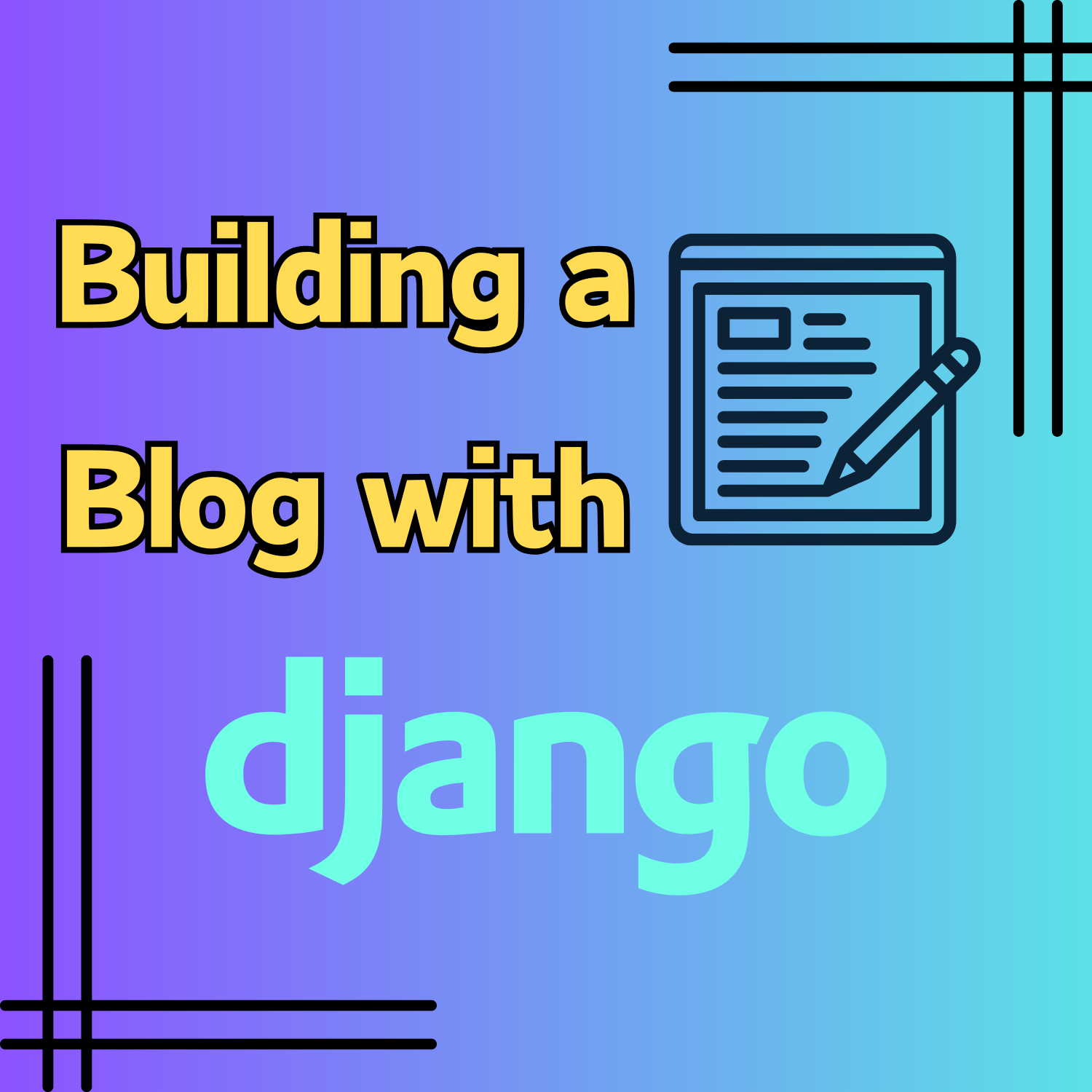
In the ever-evolving landscape of web development, Python's Django framework has emerged as a powerful tool for creating robust and scalable web applications. Among its many applications, Django is particularly well-suited for building blogs due to its flexibility, security features, and the rapid development it enables. In this comprehensive guide, we'll walk through the process of creating a fully functional blog using Python and Django.
Understanding Django
Before diving into the nitty-gritty of building a blog, it's essential to have a basic understanding of Django and its components.
What is Django?
Django is a high-level, open-source web framework written in Python. It follows the Model-View-Controller (MVC) architectural pattern but refers to it as the Model-View-Template (MVT) pattern. Django aims to simplify the development of complex, database-driven websites by providing a clean and pragmatic design.
Key Components of Django
-
Models: Models define the structure of your database and are used to create, read, update, and delete records.
-
Views: Views handle the user interface and business logic. They receive requests, process data, and return responses.
-
Templates: Templates are responsible for generating HTML dynamically. They allow you to separate the design from the logic.
-
URLs: The URL configuration determines how URLs are mapped to views. It acts as a roadmap for your application.
-
Forms: Forms handle user input and can be used for creating, updating, and deleting data.
-
Admin Panel: Django provides a built-in admin panel for managing models and their data.
Setting Up the Environment
To get started, make sure you have Python and Django installed on your machine. You can install Django using the following command:
pip install django
Once installed, create a new Django project using:
django-admin startproject myblog
Navigate into your project directory:
cd myblog
Designing the Data Model
The foundation of any blog is its data model. In Django, data models are created using Python classes. Open the models.py
file in your blog
app and define a simple model for blog posts:
# blog/models.py
from django.db import models
from django.utils import timezone
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
pub_date = models.DateTimeField(default=timezone.now)
def __str__(self):
return self.title
This model defines a Post
with a title, content, and publication date. The __str__
method is used to represent the object as a string in the admin interface.
After defining the model, create and apply migrations:
python manage.py makemigrations
python manage.py migrate
Building Views and Templates
With the data model in place, it's time to create views and templates for displaying blog content. In your blog
app, open the views.py
file and define a view to fetch and display all blog posts:
# blog/views.py
from django.shortcuts import render
from .models import Post
def post_list(request):
posts = Post.objects.all()
return render(request, 'blog/post_list.html', {'posts': posts})
Next, create a templates
folder inside the blog
app and add a new file named post_list.html
:
<!-- blog/templates/blog/post_list.html -->
<!DOCTYPE html>
<html>
<head>
<title>My Blog</title>
</head>
<body>
<h1>My Blog</h1>
{% for post in posts %}
<div>
<h2>{{ post.title }}</h2>
<p>{{ post.content }}</p>
<p>Published on: {{ post.pub_date }}</p>
</div>
{% endfor %}
</body>
</html>
This template uses Django's template language to iterate over the list of posts and display their titles, content, and publication dates.
Now, create a URL pattern to map the post_list
view. In your blog
app, create a new file named urls.py
:
# blog/urls.py
from django.urls import path
from .views import post_list
urlpatterns = [
path('', post_list, name='post_list'),
]
Include these URLs in the main urls.py
file of your project:
# myblog/urls.py
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('blog.urls')),
]
Now, when you run your development server (python manage.py runserver
), you should be able to access your blog at http://127.0.0.1:8000/
and see a list of posts.
Enhancing the Blog
While the basic blog is functional, there are several enhancements we can make to improve its functionality and appearance.
Adding Detail Views
Let's create a detail view for individual blog posts. Update your views.py
file:
# blog/views.py
from django.shortcuts import render, get_object_or_404
from .models import Post
def post_list(request):
posts = Post.objects.all()
return render(request, 'blog/post_list.html', {'posts': posts})
def post_detail(request, pk):
post = get_object_or_404(Post, pk=pk)
return render(request, 'blog/post_detail.html', {'post': post})
Now, create a new template post_detail.html
:
<!-- blog/templates/blog/post_detail.html -->
<!DOCTYPE html>
<html>
<head>
<title>{{ post.title }}</title>
</head>
<body>
<h1>{{ post.title }}</h1>
<p>{{ post.content }}</p>
<p>Published on: {{ post.pub_date }}</p>
</body>
</html>
Update the urls.py
file in the blog
app:
# blog/urls.py
from django.urls import path
from .views import post_list, post_detail
urlpatterns = [
path('', post_list, name='post_list'),
path('post/<int:pk>/', post_detail, name='post_detail'),
]
Now, clicking on a post's title in the list view should take you to the detailed view.
Integrating Bootstrap for Styling
Improve the visual appeal of your blog by integrating Bootstrap, a popular front-end framework. Download and include Bootstrap in your project. You can use a CDN or download the files and include them locally.
Create a static
folder in your blog
app and add a css
folder inside it. Download the Bootstrap CSS file and save it as bootstrap.min.css
in the css
folder.
Next, update your base.html
file to include Bootstrap:
<!-- blog/templates/blog/base.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{% block title %}My Blog{% endblock %}</title>
<link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}">
</head>
<body>
<div class="container">
{% block content %}{% endblock %}
</div>
</body>
</html>
This modification sets up a basic HTML structure and includes the Bootstrap stylesheet. The {% block %}
tags indicate areas that can be overridden in child templates.
Now, update your post_list.html
and post_detail.html
templates to extend the base.html
template:
<!-- blog/templates/blog/post_list.html -->
{% extends 'blog/base.html' %}
{% block content %}
<h1>My Blog</h1>
{% for post in posts %}
<div class="card my-3">
<div class="card-body">
<h2 class="card-title">{{ post.title }}</h2>
<p class="card-text">{{ post.content }}</p>
<p class="card-text"><small class="text-muted">Published on: {{ post.pub_date }}</small></p>
<a href="{% url 'post_detail' pk=post.pk %}" class="btn btn-primary">Read More</a>
</div>
</div>
{% endfor %}
{% endblock %}
<!-- blog/templates/blog/post_detail.html -->
{% extends 'blog/base.html' %}
{% block content %}
<h1>{{ post.title }}</h1>
<p>{{ post.content }}</p>
<p><small class="text-muted">Published on: {{ post.pub_date }}</small></p>
{% endblock %}
These modifications apply Bootstrap styles to your blog, making it more visually appealing.
Implementing User Authentication
To allow users to create and manage their blog posts, implement user authentication. Django provides built-in authentication views and forms. Update your urls.py
file in the blog
app:
# blog/urls.py
from django.urls import path
from .views import post_list, post_detail, post_create, post_edit, post_delete
from django.contrib.auth import views as auth_views
urlpatterns = [
path('', post_list, name='post_list'),
path('post/<int:pk>/', post_detail, name='post_detail'),
path('post/new/', post_create, name='post_create'),
path('post/<int:pk>/edit/', post_edit, name='post_edit'),
path('post/<int:pk>/delete/', post_delete, name='post_delete'),
path('login/', auth_views.LoginView.as_view(), name='login'),
path('logout/', auth_views.LogoutView.as_view(), name='logout'),
path('signup/', signup, name='signup'),
]
Here, new views (post_create
, post_edit
, post_delete
) are added for creating, editing, and deleting blog posts. Additionally, authentication views for login and logout are included.
Create corresponding templates for these new views, and don't forget to update the navigation in your base.html
file to reflect the authentication status:
<!-- blog/templates/blog/base.html -->
<!-- ... (previous content) ... -->
<body>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="{% url 'post_list' %}">My Blog</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
{% if user.is_authenticated %}
<li class="nav-item">
<a class="nav-link" href="{% url 'post_create' %}">New Post</a>
</li>
<li class="nav-item">
<a class="nav-link" href="{% url 'logout' %}">Logout</a>
</li>
{% else %}
<li class="nav-item">
<a class="nav-link" href="{% url 'login' %}">Login</a>
</li>
<li class="nav-item">
<a class="nav-link" href="{% url 'signup' %}">Sign Up</a>
</li>
{% endif %}
</ul>
</div>
</nav>
<div class="container">
{% block content %}{% endblock %}
</div>
</body>
</html>
Update your views.py
file in the blog
app to include the new views and authentication:
# blog/views.py
from django.shortcuts import render, get_object_or_404, redirect
from django.contrib.auth.decorators import login_required
from django.utils import timezone
from .models import Post
from .forms import PostForm
def post_list(request):
posts = Post.objects.all()
return render(request, 'blog/post_list.html', {'posts': posts})
def post_detail(request, pk):
post = get_object_or_404(Post, pk=pk)
return render(request, 'blog/post_detail.html', {'post': post})
@login_required
def post_create(request):
if request.method == 'POST':
form = PostForm(request.POST)
if form.is_valid():
post = form.save(commit=False)
post.author = request.user
post.pub_date = timezone.now()
post.save()
return redirect('post_detail', pk=post.pk)
else:
form = PostForm()
return render(request, 'blog/post_form.html', {'form': form})
@login_required
def post_edit(request, pk):
post = get_object_or_404(Post, pk=pk)
if request.method == 'POST':
form = PostForm(request.POST, instance=post)
if form.is_valid():
post = form.save(commit=False)
post.author = request.user
post.pub_date = timezone.now()
post.save()
return redirect('post_detail', pk=post.pk)
else:
form = PostForm(instance=post)
return render(request, 'blog/post_form.html', {'form': form})
@login_required
def post_delete(request, pk):
post = get_object_or_404(Post, pk=pk)
post.delete()
return redirect('post_list')
Finally, create a forms.py
file in the blog
app to define a form for creating and editing blog posts:
# blog/forms.py
from django import forms
from .models import Post
class PostForm(forms.ModelForm):
class Meta:
model = Post
fields = ['title', 'content']
Now, users can register, log in, and create, edit, or delete their blog posts.
Building a blog with Python and Django is a rewarding journey that allows you to explore various aspects of web development, from data modeling to user authentication. This guide has walked you through the process of setting up a Django project, designing a data model, creating views and templates, enhancing the blog's appearance with Bootstrap, and implementing user authentication.
As you continue to develop your blog, consider exploring additional features and optimizations, such as adding comments, implementing search functionality, or deploying your blog to a hosting platform. Django's rich ecosystem and vibrant community provide ample resources for expanding and refining your web development skills.