Unleashing The Power Of Python Django: A Comprehensive Guide To Web Development
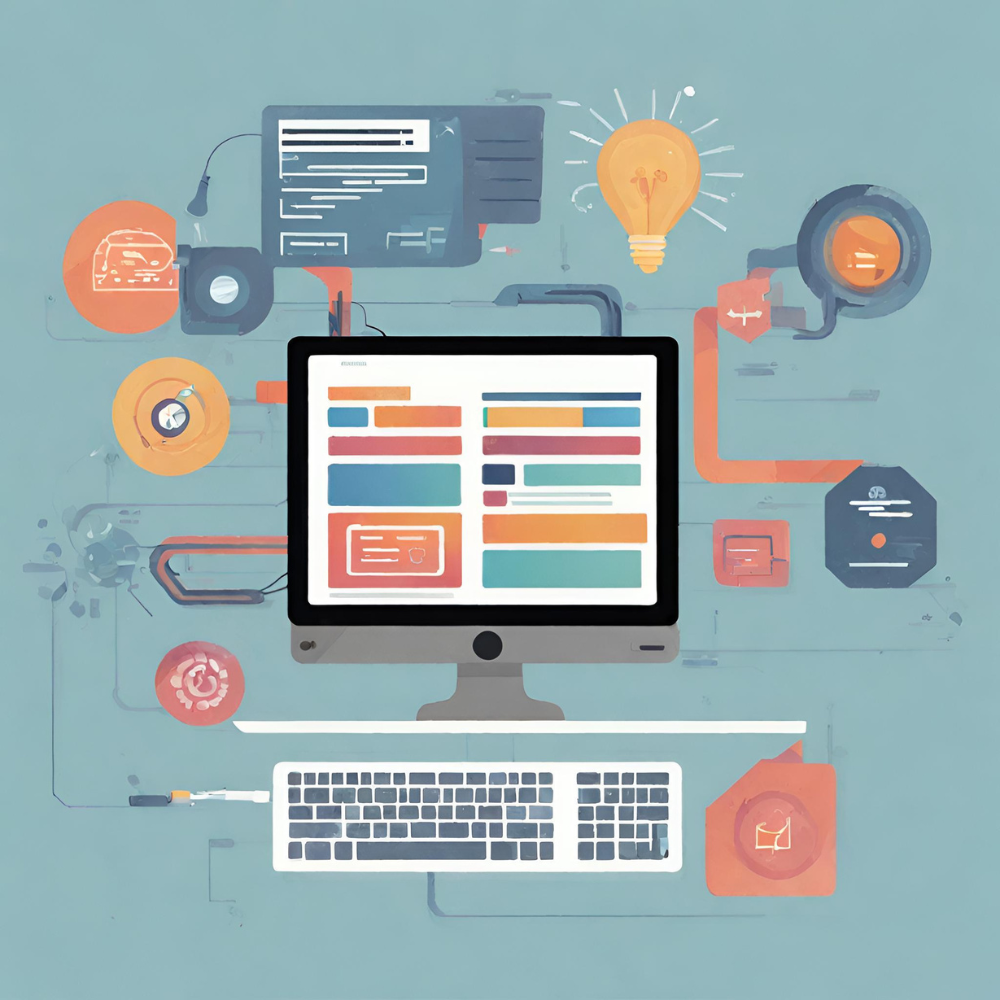
In the ever-evolving landscape of web development, Python Django stands tall as a robust and versatile framework. With its elegant design, scalability, and rapid development capabilities, Django has gained immense popularity among developers worldwide. In this comprehensive guide, we will delve deep into the world of Python Django, exploring its key features, and advantages, and providing practical examples to demonstrate its prowess.
Understanding Python Django:
The MVC architectural paradigm is used by the open-source Django web framework for Python. Its primary goal is to make web development simpler by emphasizing reusability, minimal code, rapid development, and the "Don't Repeat Yourself" (DRY) concept. Django's versatility allows programmers to build a wide range of web applications, from simple web pages to complex, data-driven structures.
Key Features of Django:
- Object-Relational Mapping (ORM): Django’s ORM system enables seamless interaction with databases, allowing developers to work with database models using Python code, eliminating the need for complex SQL queries.
# Example of Django Model
from django.db import models
class UserProfile(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
-
Admin Interface: Django comes with a built-in admin interface, providing a hassle-free way to manage application data. Developers can customize the admin panel according to the application's requirements.
-
URL Mapping: Django's URL routing system simplifies the mapping of URLs to views, making it easy to create clean, SEO-friendly URLs for web applications.
# Example of URL Mapping in Django
from django.urls import path
from . import views
urlpatterns = [
path('home/', views.home, name='home'),
]
- Template Engine: Django’s template engine allows developers to create dynamic web pages by embedding Python-like code within HTML templates, facilitating the creation of visually appealing and interactive interfaces.
<!-- Example Django Template -->
<h1>Hello, {{ user.username }}!</h1>
-
Security Features: Django provides built-in security features such as protection against SQL injection, cross-site scripting (XSS) attacks, and clickjacking, ensuring the development of secure web applications.
Advantages of Using Django:
- Rapid Development: Django's high-level abstractions and ease of use enable programmers to quickly and efficiently create fully functional web applications, significantly reducing the time required for development.
- Scalability: Django's scalable architecture allows applications to grow with the user base without breaking when traffic loads are high.
- Community and Documentation: Django provides a wealth of resources, including plugins, tutorials, and tools, to help developers enhance their projects. It has extensive documentation and a thriving community as well.
- Versatility: Django's adaptability and capacity to be integrated with various technologies and outside services make it a great choice for a variety of web development needs.
- Built-in Features: Django comes with a ton of built-in features, such as form handling, that make development easier and reduce the need for external libraries.
Practical Example: Building a Simple Django Web Application
Let's illustrate Django's power by building a basic web application that displays a list of products.
-
Installation: First, ensure you have Python installed. Then, install Django using pip:
pip install django
- Create a Django Project and App: Create a new Django project and a new app within the project.
django-admin startproject productcatalog
cd productcatalog
python manage.py startapp products
- Define Models: Define the Product model in the
products/models.py
file.
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
- Migrate Database: Create database tables based on the defined models.
python manage.py makemigrations
python manage.py migrate
- Create Views: Define a view to fetch products from the database and render them.
from django.shortcuts import render
from .models import Product
def product_list(request):
products = Product.objects.all()
return render(request, 'products/product_list.html', {'products': products})
- Create Templates: Create an HTML template to display the list of products. Save this file as
product_list.html
in theproducts/templates/products/
directory.
<!DOCTYPE html>
<html>
<head>
<title>Product Catalog</title>
</head>
<body>
<h1>Product Catalog</h1>
<ul>
{% for product in products %}
<li>{{ product.name }} - ${{ product.price }}</li>
{% empty %}
<li>No products available</li>
{% endfor %}
</ul>
</body>
</html>
- Configure URLs: Configure URL patterns to map the view to a URL.
from django.urls import path
from .views import product_list
urlpatterns = [
path('products/', product_list, name='product_list'),
]
- Run the Development Server: Start the Django development server and visit
http://localhost:8000/products/
to see the list of products.
python manage.py runserver
To sum up:
Developers can create dependable, scalable, and secure web applications with ease using Python Django. It makes a great choice for web development projects thanks to its extensive feature set, user-friendly interface, and welcoming community. If web developers follow Django's tenets and apply its features with practical examples, they can experiment with a wide range of possibilities. Regardless of the level of development experience, learning Django opens the door to creating innovative and dynamic web applications that meet the demands of the modern digital landscape. Explore Django now to see how it will affect your web development projects. Enjoy yourself while you code!