Task 20 To Task 23 Of 45 Assignments (Giaic)
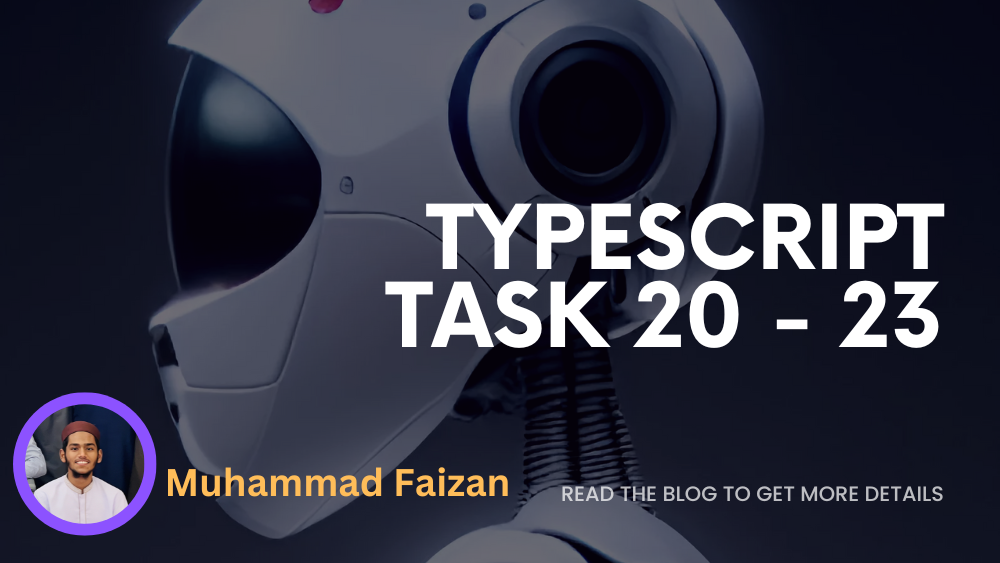
Typescript Assignment Solutions: Tasks 20-23 Completed!
In this article, we share the solutions to Typescript assignment questions 20-23. If you're stuck or need a reference, this is the perfect resource for you! We've provided clear and concise code snippets, along with explanations to help you understand the logic and implementation.
Whether you're a student working on your assignments or a developer looking for a quick reference, this article has got you covered. Take a look and learn how to tackle these Typescript challenges with ease!
Please try to do it yourself first!
# 20 Think of something you could store in a array. For example, you could make a list of mountains, rivers, countries, cities, languages, or anything else you’d like. Write a program that creates a list containing these items.
let beautiful_places:string[] = ['Mount Everest','K2', 'Murree', 'River Jehlum', 'Islamabad', 'Gilgit']
console.log('Some beautiful places to visit in Pakistan are: ',beautiful_places)
# 21 Think of something you could store in a TypeScript Object. Write a program that creates Objects containing these items.
let user: {name: string, institute: string, age: number, gender: 'Male' | 'Female'} = {
name: 'Muhammad Faizan',
institute: 'GIAIC',
age: 17,
gender: 'Male',
}
console.log(user)
# 22 Intentional Error: Try to produce an array index error in one of your programs. Correct the error before finishing.
let rivers = ['Jehlum','Ravi']
console.log(rivers[2]) // Asking for out of index value, which will return UNDEFINED
console.log(rivers[0]) // Now asking for correct index value
# 23 Conditional Tests: Write a series of conditional tests. Predict the results of each test.
// Test 01
let food = 'biryani'
console.log("Is food == biryani or not? 'I think YES!' ")
console.log(food == 'biryani')
console.log(`It returns ${food == 'biryani'} which means food is biryani!`)
// Test 02
let car = 'corolla'
console.log("Is car == corolla or not? 'I think YES!' ")
console.log(car == 'corolla')
console.log(`It returns ${car == 'corolla'} which means car is corolla!`)
// Test 03
let laptop = 'Dell'
console.log("Is laptop == Dell or not? 'I think YES!' ")
console.log(laptop == 'Dell')
console.log(`It returns ${laptop == 'Dell'} which means laptop is Dell!`)
// Test 04
let mobile = 'oppo'
console.log("Is mobile == oppo or not? 'I think YES!' ")
console.log(mobile == 'oppo')
console.log(`It returns ${mobile == 'oppo'} which means mobile is oppo!`)
// Test 05
let pen = 'piano'
console.log("Is pen == piano or not? 'I think YES!' ")
console.log(pen == 'piano')
console.log(`It returns ${pen == 'piano'} which means pen is piano!`)
// Test 06
let dinner = 'pasta'
console.log("Is dinner == biryani or not? 'I think NO!' ")
console.log(dinner == 'biryani')
console.log(`It returns ${dinner == 'biryani'} which means dinner is not biryani!`)
// Test 07
let bike = 'unique'
console.log("Is bike == Crown or not? 'I think NO!' ")
console.log(bike == 'Crown')
console.log(`It returns ${bike == 'Crown'} which means bike is not Crown!`)
// Test 08
let mouse = 'Dell'
console.log("Is mouse == hp or not? 'I think NO!'")
console.log(mouse == 'hp')
console.log(`It returns ${mouse == 'hp'} which means mouse is not of hp!`)
// Test 09
let fruit = 'apple'
console.log("Is fruit == Mango or not? 'I think NO!' ")
console.log(fruit == 'Mango')
console.log(`It returns ${fruit == 'Mango'} which means fruit is not Mango!`)
// Test 10
let institute = 'GIAIC'
console.log("Is institute == PIAIC or not? 'I think NO!' ")
console.log(institute == 'PIAIC')
console.log(`It returns ${institute == 'PIAIC'} which means institute is not PIAIC!`)
Important Links
IT Mate Pakistan Playlist For Typescript