Empowering Digital Connections: Building A Django Url Shortener
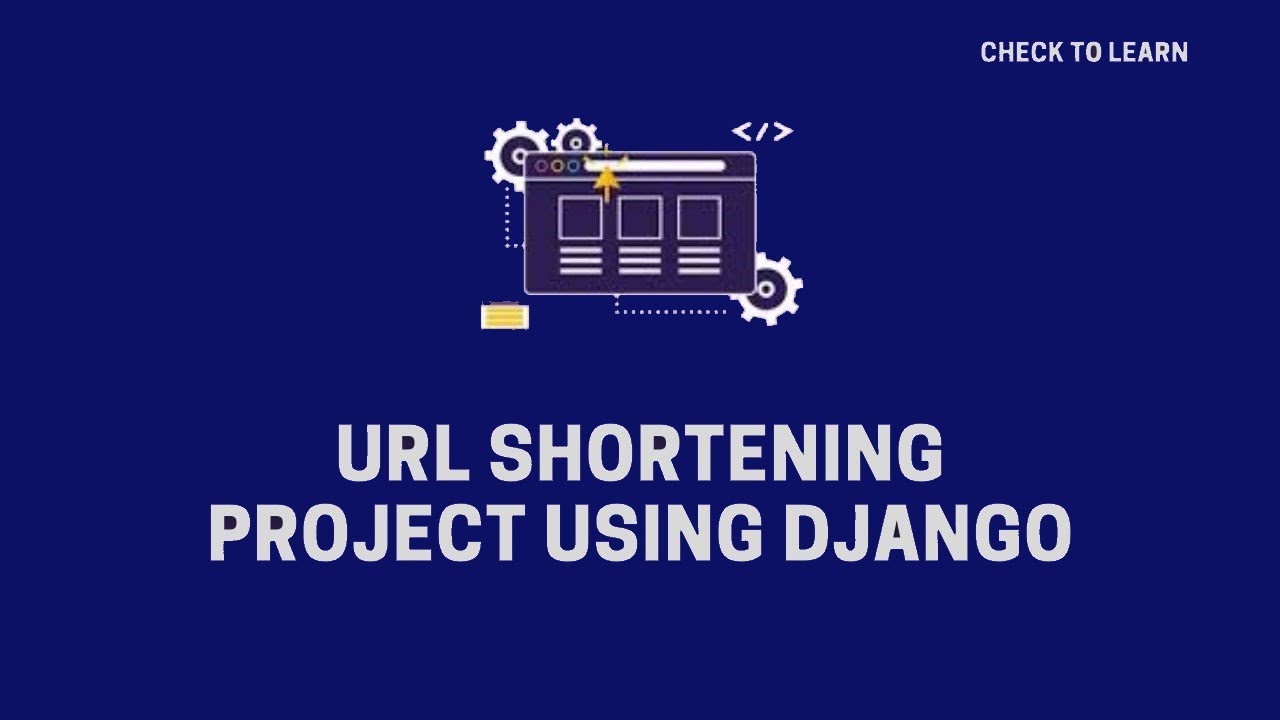
In the vast expanse of the internet, where every click leads to a new discovery, the length of a URL can make a significant difference in user experience. Long, unwieldy URLs can be cumbersome and aesthetically unpleasing, leading to the rise in popularity of URL shorteners. These tools not only condense lengthy web addresses but also enhance the efficiency of sharing and tracking links. In this article, we will explore the world of Django URL shorteners, shedding light on how they work and how you can build your own efficient and customizable URL shortener using Django, one of the most robust web frameworks in the world of web development.
Understanding the Basics: What is a URL Shortener?
A URL shortener is a web service that transforms a long URL into a shorter, more manageable version. This condensed URL redirects users to the original, longer URL when accessed. This technology is widely used in various scenarios, such as social media sharing, email marketing campaigns, and even academic research. Its primary goal is to simplify complex URLs, making them easier to share, type, and remember.
Why Choose Django for a URL Shortener?
Django, a high-level Python web framework, is renowned for its simplicity, scalability, and versatility. Leveraging Django for building a URL shortener provides a robust foundation. Its built-in features, such as object-relational mapping (ORM) and robust security protocols, ensure a streamlined development process while maintaining the integrity and security of the application.
Setting Up Your Django Project:
-
Installation: Begin by installing Django using the following pip command:
pip install django
-
Creating a Django Project: Create a new Django project using the following command:
django-admin startproject urlshortener
-
Creating a URL Shortener App: Inside your project directory, create a new app for the URL shortener:
python manage.py startapp shortener
Building the URL Shortener:
-
Creating Models: Define a model for the URL shortener in your
shortener/models.py
file:from django.db import models class ShortenedURL(models.Model): original_url = models.URLField(max_length=200) short_url = models.CharField(max_length=10, unique=True)
-
Generating Short URLs: Implement a function to generate short URLs, and override the
save
method in your model:import string import random def generate_short_url(): characters = string.ascii_letters + string.digits short_url = ''.join(random.choice(characters) for _ in range(6)) return short_url class ShortenedURL(models.Model): # ... (previous fields) def save(self, *args, **kwargs): if not self.short_url: self.short_url = generate_short_url() super(ShortenedURL, self).save(*args, **kwargs)
-
Creating Views and Templates: Implement views for handling URL shortening and redirection in your
shortener/views.py
file. Create corresponding templates for user interface elements. -
Setting Up URL Patterns: Define URL patterns in your
shortener/urls.py
file to route requests to appropriate views. -
Migrating and Running the Application: Apply migrations and run the development server to see your URL shortener in action:
python manage.py makemigrations python manage.py migrate python manage.py runserver
Enhancing the URL Shortener:
-
Adding User Authentication: Implement user authentication to provide secure access to URL-shortening services, allowing users to manage their shortened links.
-
Implementing Analytics: Integrate tracking mechanisms to gather data on link clicks and user engagement, enabling users to monitor the performance of their shortened URLs.
-
Implementing Custom Short URLs: Allow users to create custom short URLs, enhancing personalization and brand representation.
-
Implementing Expiry Mechanism: Add an expiry mechanism to automatically deactivate short URLs after a specified period, ensuring that outdated links do not redirect users.
Conclusion:
In conclusion, building a Django URL shortener not only provides a practical solution for managing long URLs but also serves as an excellent exercise for mastering Django's capabilities. Through this project, developers can delve into various aspects of web development, including database modeling, user authentication, and view routing.
The simplicity and flexibility of Django make it an ideal choice for this endeavor, empowering developers to create a fully functional and efficient URL shortener while gaining valuable experience in building web applications. By understanding the fundamentals of URL shortening and leveraging Django's powerful features, developers can contribute to the digital landscape by offering a seamless, user-friendly experience for sharing and accessing online content.