Creating A Comment System In Django: A Step-By-Step Guide
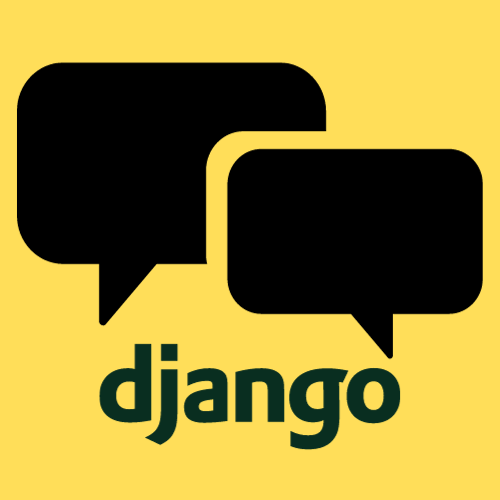
Introduction
In the world of web development, interactivity and engagement are key components of a successful website. One way to enhance user engagement is by adding a comment system to your website. In this tutorial, we will explore how to create a robust and efficient comment system using Django, a high-level Python web framework. By the end of this guide, you will have a fully functional comment system that you can integrate into your Django-based web applications.
Prerequisites
Before we start building our comment system, make sure you have the following prerequisites:
-
Python and Django Installed: Ensure you have Python installed on your system. You can download it from the official Python website (python.org). Additionally, install Django using the following command:
pip install django
-
Basic Understanding of Django: Familiarity with Django concepts such as models, views, and templates will be beneficial.
Step 1: Set Up a Django Project
Begin by creating a new Django project using the following command:
django-admin startproject comment_system
Navigate to the project directory:
cd comment_system
Step 2: Create a Django App
Next, create a Django app within your project. For our comment system, let's name the app "comments."
python manage.py startapp comments
Add the newly created app to your project INSTALLED_APPS
in the settings.py
file.
Step 3: Design the Comment Model
Define the comment model in the models.py
file inside the "comments" app. For example:
from django.db import models
class Comment(models.Model):
author = models.CharField(max_length=100)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.author
Step 4: Create Comment Forms
Create a Django form to handle comment input. In the forms.py
file inside the "comments" app:
from django import forms
from .models import Comment
class CommentForm(forms.ModelForm):
class Meta:
model = Comment
fields = ['author', 'content']
Step 5: Create Views and Templates
Create views for displaying and handling comments. Design corresponding templates for user interaction.
- In
views.py
:
from django.shortcuts import render
from .forms import CommentForm
from .models import Comment
def comments(request):
comments = Comment.objects.all()
if request.method == 'POST':
form = CommentForm(request.POST)
if form.is_valid():
form.save()
else:
form = CommentForm()
return render(request, 'comments/comments.html', {'comments': comments, 'form': form})
- Create a
comments.html
template inside the "templates" folder in the "comments" app to display comments and the comment form.
Step 6: Configure URLs
Configure the URLs to handle comment-related requests. In the urls.py
file inside the "comments" app:
from django.urls import path
from . import views
urlpatterns = [
path('', views.comments, name='comments'),
]
Include the app's URLs in the project's urls.py
file.
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls), path('comments/', include('comments.urls')),
]
Step 7: Run the Development Server
Finally, run the development server using the following command:
python manage.py runserver
Visit http://localhost:8000/comments/ in your browser to access the comment system. Users can now view and submit comments seamlessly.
Conclusion
In this tutorial, you've learned how to create a comment system in Django from scratch. By following these steps, you've set up a foundation for user engagement on your website. Remember, this is a basic implementation, and you can further enhance the system by adding features like comment moderation, user authentication, and real-time updates. Happy coding!