Building A Png To Jpg Converter With Html, Css, And Javascript
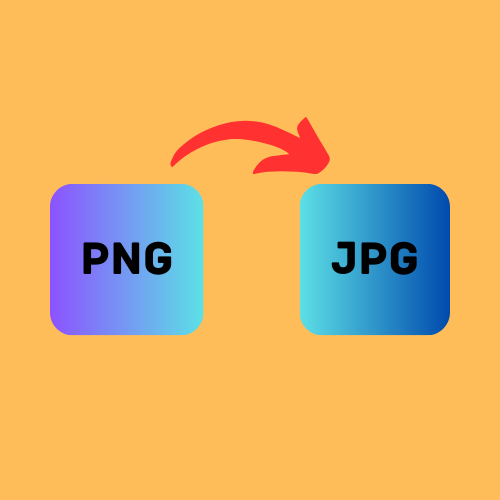
In the dynamic world of web development, creating interactive and user-friendly tools enhances the overall browsing experience. One such tool that can be crafted with the trio of HTML, CSS, and JavaScript is a PNG to JPG converter. This article will guide you through the process of building a simple yet effective converter, allowing users to seamlessly transform PNG images into the widely supported JPG format.
1. Setting the Stage: HTML Structure
To start, let's lay the foundation with HTML. Create the basic structure of your webpage, incorporating the necessary elements like buttons and input fields. The user interface is pivotal, so ensure a clean and intuitive layout.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PNG to JPG Converter</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>PNG to JPG Converter</h1>
<p>Select a PNG file, convert it to JPG, and download the converted image.</p>
<label for="fileInput" class="custom-file-upload">
Choose File
</label>
<input type="file" id="fileInput" accept=".png">
<button onclick="convertToJpg()">Convert to JPG</button>
<a id="downloadLink" style="display:none" download="converted_image.jpg">Download JPG</a>
</div>
<script src="script.js"></script>
</body>
</html>
2. Styling for Appeal: CSS Enhancement
A visually appealing interface is crucial for user engagement. Enhance the aesthetics of your converter using CSS. Style the components to provide a modern and intuitive look.
body {
font-family: 'Arial', sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.container {
text-align: center;
background-color: #fff;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
color: #333;
}
p {
color: #666;
margin-bottom: 20px;
}
.custom-file-upload {
display: inline-block;
padding: 8px 16px;
cursor: pointer;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
transition: background-color 0.3s;
}
.custom-file-upload:hover {
background-color: #45a049;
}
input[type="file"] {
display: none;
}
button {
background-color: #4caf50;
color: #fff;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
border: none;
border-radius: 4px;
transition: background-color 0.3s;
}
button:hover {
background-color: #45a049;
}
a {
display: none;
color: #fff;
background-color: #007bff;
padding: 10px 20px;
font-size: 16px;
text-decoration: none;
border-radius: 4px;
}
a:hover {
background-color: #0056b3;
}
3. Dynamic Functionality: JavaScript Magic
Now, let's infuse life into our converter using JavaScript. The core functionality involves reading a PNG file, converting it to a JPG data URL, and allowing users to download the converted image.
function convertToJpg() {
const fileInput = document.getElementById('fileInput');
const downloadLink = document.getElementById('downloadLink');
// Check if a file is selected
if (fileInput.files.length > 0) {
const pngFile = fileInput.files[0];
// Create a FileReader to read the PNG file
const reader = new FileReader();
reader.onload = function (event) {
const pngDataUrl = event.target.result;
// Create an Image element to load the PNG data URL
const img = new Image();
img.onload = function () {
// Create a canvas to draw the PNG image
const canvas = document.createElement('canvas');
canvas.width = img.width;
canvas.height = img.height;
const ctx = canvas.getContext('2d');
ctx.drawImage(img, 0, 0);
// Convert the canvas to a data URL with JPEG format
const jpgDataUrl = canvas.toDataURL('image/jpeg', 0.8);
// Set the download link href and display it
downloadLink.href = jpgDataUrl;
downloadLink.style.display = 'inline';
};
img.src = pngDataUrl;
};
// Read the PNG file as a data URL
reader.readAsDataURL(pngFile);
} else {
alert('Please select a PNG file.');
}
}
Building a PNG to JPG converter with HTML, CSS, and JavaScript demonstrates the seamless integration of these web technologies. The combination of an intuitive user interface, visually appealing design, and dynamic functionality creates a powerful tool that simplifies the image conversion process for users. As you embark on your web development journey, remember that creativity and functionality go hand in hand, making your projects both useful and visually engaging.